Streaming ChatGPT Responses in Python
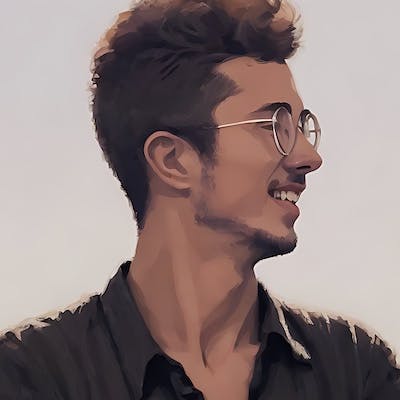
Paul Berthelot / April 04, 2023
2 min read • –––
Introduction
Hey there! In this blog post, I'm going to show you how to use ChatGPT in Python and stream responses, making your interaction with the AI more engaging and real-time. We'll be using OpenAI's API and the awesome GPT-3.5-turbo model.
Requirements
Before we start, make sure you've got these things ready:
- An OpenAI API key. Sign up on the OpenAI platform if you don't have one.
- Python installed on your computer.
- The OpenAI Python library. Just run
pip install openai
.
Alright, let's dive into the code!
Step 1: Import the necessary libraries
import sys
import openai
Step 2: Set up your API key
Don't forget to set up your API key before making any API calls.
openai.api_key = "your-api-key-here"
Step 3: Create the generate_text function
Check out this generate_text function I made. It takes a list of messages, sends them to the ChatGPT API, and streams the response.
def generate_text(messages):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages,
stream=True,
)
collected_messages = ""
for message in response:
for chunk in message:
chunk_message = chunk['choices'][0]['delta']
if hasattr(chunk_message, 'content'):
sys.stdout.write(chunk_message['content'])
sys.stdout.flush()
return collected_messages
Step 4: Set up the messages and call the generate_text function
Now let's set up our messages and call the generate_text function to see the magic happen!
messages = [
{"role": "system", "content": "You are Eko, a helpful assistant. You are talking to a user named Paul."},
{"role": "user", "content": "Hello Eko, write me a recipe with eggs and bacon today!"}
]
answerAI = generate_text(messages)
And there you have it! Follow these steps, and you'll be using ChatGPT in Python to generate and stream responses in no time. The generate_text function will keep streaming the response from the ChatGPT API, allowing you to display it as it happens. Enjoy!
Here is the final code.
import sys
import openai
openai.api_key = "your-api-key-here"
def generate_text(messages):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages,
stream=True),
collected_messages = ""
for message in response:
for chunk in message:
chunk_message = chunk['choices'][0]['delta']
if hasattr(chunk_message, 'content'):
sys.stdout.write(chunk_message['content'])
sys.stdout.flush()
return collected_messages
messages = [
{"role": "system", "content": "You are Eko, a helpful assistant. You are talking to a user named Paul."}
{"role": "user", "content": "Hello Eko, write me a recipe with eggs and bacon today!"}
]
answerAI = generate_text(messages)
Subscribe to the newsletter
Get emails from me about web development, tech trends, and advices for founders.
24 subscribers