Using Google APIs with Node.js and uploading a YouTube video
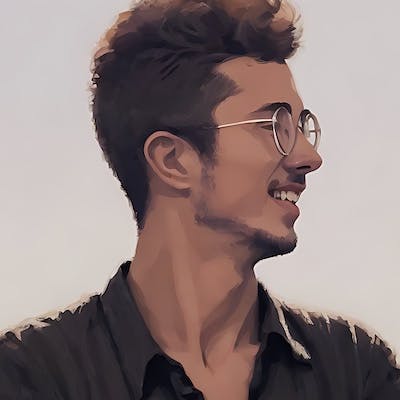
Paul Berthelot / January 09, 2023
7 min read • –––
Why using Google APIs?
Are you tired of trying to build a feature or integrate with a service on your own, only to find that it takes way longer than expected and doesn't work as well as you hoped? If so, you may want to consider using Google APIs.
Use cases for using Google APIs
-
YouTube API: You can use the YouTube API to access YouTube videos, channels, playlists and streaming. This can be useful for creating a video website or application, or for adding video functionality to an existing site or app.
-
Google Maps API: You can use the Google Maps API to add maps, location markers, and other location-based functionality to your website or application. This can be useful for creating a real estate website, a travel app, or any other type of application that involves mapping.
-
Google AdSense API: You can use the Google AdSense API to manage your AdSense account and access reporting data. This can be useful for automating tasks related to AdSense, such as generating reports or modifying ad settings.
-
Google Calendar API: You can use the Google Calendar API to access and manage events and calendars. This can be useful for creating a calendar application or integrating calendar functionality into an existing application.
-
Google Drive API: You can use the Google Drive API to access and manage files stored in Google Drive. This can be useful for creating a file storage and collaboration application, or for integrating Google Drive functionality into an existing application.
These are just a few examples of the many Google APIs that are available. There are many more APIs available for different purposes, such as the Google Analytics API, the Google Sheets API, and the Google Translate API, to name a few.
Using Google APIs can also help you leverage the power and reach of Google's vast network. For example, if you use the Google Maps API, you can easily add maps and location-based functionality to your application, taking advantage of the millions of users who already use Google Maps.
Another benefit of using Google APIs is that they are often free to use (at least up to a certain usage limit). This can be a huge advantage, especially if you are working on a small project or a startup with a limited budget. Even if you do have to pay for an API, the cost is often well worth it when you consider the time and effort it would take to build something similar on your own.
Overall, using Google APIs can be a great way to save time and effort, and take advantage of the reliability and power of Google's platform. So next time you're looking to add a new feature or integrate with a service, consider using a Google API to get the job done.
How to use Google API
-
First you need to have a google account. Then you can go to your developer dashboard where you will create and set up your projects.
-
Create a project and go to the dashboard of your project.
-
Now you can activate the api you wants for you project. Here I will activate the Youtube Data API to search and upload videos.
-
The last step is to set up authorization to your project to access the activated api from your node.js script. Depending of your use case, you will only want an api key or a complete oAuth2 authentication.
- API Key: If you want to use the API on a global scope (not linked to a user), you will only need an API Key. After creating your API Key, paste it in a node.js file that we will use in a minute.
- OAuth2: If you want to use the API that will use the user account, you will need to set up a OAuth2 authorization. First create a OAuth consent screen, you will need to add specific scopes (authorizations) that the user will consent when they sign-in to your application. For now, don't add scopes, we will add them later. Then create a OAuth 2.0 client ID, you will need to add a redirection uri, you can put http://localhost:8080/myendpoint. Download the json authorization file at the end of the creation of your client id. If you don't know the basics of OAuth2 authorization, you can learn more here.
-
Now it's time to create our nodejs script to use our activated google api. We will write a script to search videos on youtube first. Each API of google (Youtube, Google maps...) provides a REST API. You can find all the API in the Google APIs Explorer. To search and upload videos on youtube, we will use the YouTube Data API v3.
The endpoint to search videos is
https://www.googleapis.com/...
...youtube/v3/search
.
You can find the documentation here.
const fetch = require('node-fetch');
async function main() {
const searchQuery = 'Best vscode plugins';
const response = await fetch(
`https://www.googleapis.com/youtube/v3/search?key=${process.env.APIKEY}&type=video&part=snippet&q=${searchQuery}`
);
const data = await response.json();
const titles = data.items.map((item) => item.snippet.title);
console.log(titles);
}
But there is a better way to use the REST API. Google created a node.js client library for using Google APIs called googleapis. Each API endpoints are automatically generated. A small tip is that you can generate code examples with the documentation.
const fs = require('fs');
const readline = require('readline');
const { google } = require('googleapis');
async function main() {
// Authorization
const youtube = google.youtube({
version: 'v3',
auth: process.env.APIKEY,
});
// Search videos
const res = await youtube.search.list({
part: 'snippet',
q: 'Best vscode plugins',
type: 'video',
});
const titles = res.data.items.map((item) => item.snippet.title);
console.log(titles);
}
- Now it's time to write our script that let the user upload a video on his account. We will have to use the OAuth2 authorization flow. So first, here is our endpoint in the documentation. In this endpoint, there is a Authorization section where you can find the scopes needed. This example comes from the official repository of googleapis on GitHub.
const fs = require('fs');
const readline = require('readline');
const { google } = require('googleapis');
const youtube = google.youtube('v3');
const { authenticate } = require('@google-cloud/local-auth');
async function main(filePath) {
// Obtain user credentials to use for the request
const auth = await authenticate({
keyfilePath: path.join(__dirname, '../oauth2.keys.json'),
scopes: [
'https://www.googleapis.com/auth/youtube.upload',
'https://www.googleapis.com/auth/youtube',
],
});
google.options({ auth });
const fileSize = fs.statSync(filePath).size;
const res = await youtube.videos.insert(
{
part: 'id,snippet,status',
notifySubscribers: false,
requestBody: {
snippet: {
title: 'Node.js YouTube Upload Test',
description: 'Testing YouTube upload via Google APIs Node.js Client',
},
status: {
privacyStatus: 'private',
},
},
media: {
body: fs.createReadStream(filePath),
},
},
{
// Use the `onUploadProgress` event from Axios to track the
// number of bytes uploaded to this point.
onUploadProgress: (evt) => {
const progress = (evt.bytesRead / fileSize) * 100;
readline.clearLine(process.stdout, 0);
readline.cursorTo(process.stdout, 0, null);
process.stdout.write(`${Math.round(progress)}% complete`);
},
}
);
console.log('\n\n');
console.log(res.data);
return res.data;
}
main('./myvideo.mov');
Conclusion
You just learned how to use Google APIs! The last week, I have done a node.js script to stream on YouTube using the Youtube API. It is a little bit more complex but it can be a very good challenge! You will have to learn how to decode/encode a video and how to stream videos with protocols like RTMPS... Good luck!
Subscribe to the newsletter
Get emails from me about web development, tech trends, and advices for founders.
24 subscribers